Add to cart
100% Chemical-Free & Safe
Combo of Rosemary + Jojoba
Oil for Body, Face Glow & Frizzy Hair
100% Chemical-Free & Safe
100% Pure
95% experienced softer, shinier hair with reduced breakage in 2 weeks
MRP:
Original price was: ₹1,290.00.₹690.00Current price is: ₹690.00.
Tax included
<?php // Get the current product global $product; // Check if we're on a product page and have a product if (!is_product() || !$product) { return; } // Check if it's a variable product if (!$product->is_type('variable')) { return; } // Get variation data to access prices $variations = $product->get_available_variations(); $variation_prices = array(); // Find the 100ml and 200ml variations and their prices foreach ($variations as $variation) { $attributes = $variation['attributes']; // Check for size attribute if (isset($attributes['attribute_pa_size'])) { $size_value = $attributes['attribute_pa_size']; if (strpos($size_value, '100') !== false || strpos($size_value, '100-ml') !== false) { $variation_prices['100'] = $variation['display_price']; } elseif (strpos($size_value, '200') !== false || strpos($size_value, '200-ml') !== false) { $variation_prices['200'] = $variation['display_price']; } } } // Format prices with currency symbol $formatted_prices = array(); if (isset($variation_prices['100'])) { $formatted_prices['100'] = wc_price($variation_prices['100']); } if (isset($variation_prices['200'])) { $formatted_prices['200'] = wc_price($variation_prices['200']); } // Get current price (default to 100ML) $current_price = isset($formatted_prices['100']) ? $formatted_prices['100'] : ''; ?> <!-- Add a div to show the current price --> <div class="single-price-display"> <?php echo $current_price; ?> </div> <!-- Original size terms hidden but functional --> <div class="original-size-terms" style="position: absolute; opacity: 0; pointer-events: none; height: 0; overflow: hidden;"> <?php echo do_shortcode(''); ?> </div>
/* Hide the original price range in specific places */ .woocommerce-variation-price { display: none !important; } /* Price display styles */ .single-price-display { font-size: 24px; font-weight: 600; margin: 15px 0; display: block !important; } /* Make sure the 100 ML button appears selected by default */ .woocommerce div.product form.cart .button-variable-item[data-value="100-ml"], button[data-value="100-ml"], a[data-value="100-ml"], .button-wrapper [data-value="100-ml"], .size-option[data-value="100-ml"] { background-color: #333 !important; color: white !important; border-color: #333 !important; } /* Make sure both size buttons are clickable */ .button-variable-wrapper .button-variable-item, .button-wrapper button, .size-option { cursor: pointer !important; pointer-events: auto !important; } /* Remove any disabled styles */ .button-variable-item.disabled, button.disabled, .disabled { opacity: 1 !important; pointer-events: auto !important; }
document.addEventListener('DOMContentLoaded', function() { // Wait for the page to fully load setTimeout(function() { // Hide the original price range display const priceRanges = document.querySelectorAll('.price-range, .woocommerce-variation-price'); priceRanges.forEach(el => { el.style.display = 'none'; }); // Find price display element const priceDisplay = document.querySelector('.single-price-display'); // Pricing data from PHP const pricingData = { <?php if (isset($formatted_prices['100'])): ?> '100': '<?php echo addslashes($formatted_prices['100']); ?>', <?php endif; ?> <?php if (isset($formatted_prices['200'])): ?> '200': '<?php echo addslashes($formatted_prices['200']); ?>' <?php endif; ?> }; // Find size buttons/options - try multiple selectors const sizeOptions = document.querySelectorAll('.button-variable-item, [data-attribute="pa_size"] [data-value], .size-option, button[data-value]'); // Map sizes to their elements const sizeMap = {}; sizeOptions.forEach(option => { let sizeValue = option.getAttribute('data-value') || ''; if (sizeValue.includes('100')) { sizeMap['100'] = option; } else if (sizeValue.includes('200')) { sizeMap['200'] = option; } }); // Ensure the 100 ML option is selected by default if (sizeMap['100']) { // Force click the 100 ML button setTimeout(() => { try { sizeMap['100'].click(); } catch (e) { console.log('Could not click 100 ML option'); } }, 100); } // Add click handlers to update price display sizeOptions.forEach(option => { option.addEventListener('click', function() { const value = this.getAttribute('data-value') || ''; // Update styling for all buttons sizeOptions.forEach(btn => { btn.style.backgroundColor = ''; btn.style.color = ''; }); // Set styling for clicked button this.style.backgroundColor = '#333'; this.style.color = 'white'; // Update price based on selection if (value.includes('100') && pricingData['100']) { priceDisplay.innerHTML = pricingData['100']; } else if (value.includes('200') && pricingData['200']) { priceDisplay.innerHTML = pricingData['200']; } }); }); // Make sure variation form is initialized if (typeof jQuery !== 'undefined') { jQuery('form.variations_form').on('woocommerce_variation_has_changed', function() { // Get the current variation const variation = jQuery('input[name="variation_id"]').val(); if (variation) { // Check which size is selected const sizeValue = jQuery('select[name="attribute_pa_size"]').val() || ''; if (sizeValue.includes('100') && pricingData['100']) { priceDisplay.innerHTML = pricingData['100']; } else if (sizeValue.includes('200') && pricingData['200']) { priceDisplay.innerHTML = pricingData['200']; } } }); // Initialize form jQuery('form.variations_form').trigger('check_variations'); } // Create a hidden select for the size attribute if needed const variationForm = document.querySelector('.variations_form'); if (variationForm && !document.querySelector('select[name="attribute_pa_size"]')) { const sizeSelect = document.createElement('select'); sizeSelect.name = 'attribute_pa_size'; sizeSelect.style.display = 'none'; // Add options const option100 = document.createElement('option'); option100.value = '100-ml'; option100.text = '100 ML'; const option200 = document.createElement('option'); option200.value = '200-ml'; option200.text = '200 ML'; sizeSelect.appendChild(option100); sizeSelect.appendChild(option200); // Set default sizeSelect.value = '100-ml'; // Add to form variationForm.appendChild(sizeSelect); // Trigger change if (typeof jQuery !== 'undefined') { jQuery(sizeSelect).trigger('change'); } } }, 300); });
<?php // Get the current product global $product; // Check if we're on a product page and have a product if (!is_product() || !$product) { return; } // Check if it's a variable product if (!$product->is_type('variable')) { return; } // Get variation data to access prices $variations = $product->get_available_variations(); $variation_prices = array(); // Find the 100ml and 200ml variations and their prices foreach ($variations as $variation) { $attributes = $variation['attributes']; // Check for size attribute if (isset($attributes['attribute_pa_size'])) { $size_value = $attributes['attribute_pa_size']; if (strpos($size_value, '100') !== false || strpos($size_value, '100-ml') !== false) { $variation_prices['100'] = $variation['display_price']; } elseif (strpos($size_value, '200') !== false || strpos($size_value, '200-ml') !== false) { $variation_prices['200'] = $variation['display_price']; } } } // Format prices with currency symbol $formatted_prices = array(); if (isset($variation_prices['100'])) { $formatted_prices['100'] = wc_price($variation_prices['100']); } if (isset($variation_prices['200'])) { $formatted_prices['200'] = wc_price($variation_prices['200']); } // Get current price (default to 100ML) $current_price = isset($formatted_prices['100']) ? $formatted_prices['100'] : ''; ?> <!-- Add a div to show the current price --> <div class="single-price-display"> <?php echo $current_price; ?> </div> <!-- Original size terms hidden but functional --> <div class="original-size-terms" style="position: absolute; opacity: 0; pointer-events: none; height: 0; overflow: hidden;"> <?php echo do_shortcode(''); ?> </div>
/* Hide the original price range in specific places */ .woocommerce-variation-price { display: none !important; } /* Price display styles */ .single-price-display { font-size: 24px; font-weight: 600; margin: 15px 0; display: block !important; } /* Make sure the 100 ML button appears selected by default */ .woocommerce div.product form.cart .button-variable-item[data-value="100-ml"], button[data-value="100-ml"], a[data-value="100-ml"], .button-wrapper [data-value="100-ml"], .size-option[data-value="100-ml"] { background-color: #333 !important; color: white !important; border-color: #333 !important; } /* Make sure both size buttons are clickable */ .button-variable-wrapper .button-variable-item, .button-wrapper button, .size-option { cursor: pointer !important; pointer-events: auto !important; } /* Remove any disabled styles */ .button-variable-item.disabled, button.disabled, .disabled { opacity: 1 !important; pointer-events: auto !important; }
document.addEventListener('DOMContentLoaded', function() { // Wait for the page to fully load setTimeout(function() { // Hide the original price range display const priceRanges = document.querySelectorAll('.price-range, .woocommerce-variation-price'); priceRanges.forEach(el => { el.style.display = 'none'; }); // Find price display element const priceDisplay = document.querySelector('.single-price-display'); // Pricing data from PHP const pricingData = { <?php if (isset($formatted_prices['100'])): ?> '100': '<?php echo addslashes($formatted_prices['100']); ?>', <?php endif; ?> <?php if (isset($formatted_prices['200'])): ?> '200': '<?php echo addslashes($formatted_prices['200']); ?>' <?php endif; ?> }; // Find size buttons/options - try multiple selectors const sizeOptions = document.querySelectorAll('.button-variable-item, [data-attribute="pa_size"] [data-value], .size-option, button[data-value]'); // Map sizes to their elements const sizeMap = {}; sizeOptions.forEach(option => { let sizeValue = option.getAttribute('data-value') || ''; if (sizeValue.includes('100')) { sizeMap['100'] = option; } else if (sizeValue.includes('200')) { sizeMap['200'] = option; } }); // Ensure the 100 ML option is selected by default if (sizeMap['100']) { // Force click the 100 ML button setTimeout(() => { try { sizeMap['100'].click(); } catch (e) { console.log('Could not click 100 ML option'); } }, 100); } // Add click handlers to update price display sizeOptions.forEach(option => { option.addEventListener('click', function() { const value = this.getAttribute('data-value') || ''; // Update styling for all buttons sizeOptions.forEach(btn => { btn.style.backgroundColor = ''; btn.style.color = ''; }); // Set styling for clicked button this.style.backgroundColor = '#333'; this.style.color = 'white'; // Update price based on selection if (value.includes('100') && pricingData['100']) { priceDisplay.innerHTML = pricingData['100']; } else if (value.includes('200') && pricingData['200']) { priceDisplay.innerHTML = pricingData['200']; } }); }); // Make sure variation form is initialized if (typeof jQuery !== 'undefined') { jQuery('form.variations_form').on('woocommerce_variation_has_changed', function() { // Get the current variation const variation = jQuery('input[name="variation_id"]').val(); if (variation) { // Check which size is selected const sizeValue = jQuery('select[name="attribute_pa_size"]').val() || ''; if (sizeValue.includes('100') && pricingData['100']) { priceDisplay.innerHTML = pricingData['100']; } else if (sizeValue.includes('200') && pricingData['200']) { priceDisplay.innerHTML = pricingData['200']; } } }); // Initialize form jQuery('form.variations_form').trigger('check_variations'); } // Create a hidden select for the size attribute if needed const variationForm = document.querySelector('.variations_form'); if (variationForm && !document.querySelector('select[name="attribute_pa_size"]')) { const sizeSelect = document.createElement('select'); sizeSelect.name = 'attribute_pa_size'; sizeSelect.style.display = 'none'; // Add options const option100 = document.createElement('option'); option100.value = '100-ml'; option100.text = '100 ML'; const option200 = document.createElement('option'); option200.value = '200-ml'; option200.text = '200 ML'; sizeSelect.appendChild(option100); sizeSelect.appendChild(option200); // Set default sizeSelect.value = '100-ml'; // Add to form variationForm.appendChild(sizeSelect); // Trigger change if (typeof jQuery !== 'undefined') { jQuery(sizeSelect).trigger('change'); } } }, 300); });
Rosemary & Fenugreek Oil: 200ml
Jojba Oil: 100ml
Jojba Oil: 100ml
Rosemary & Fenugreek Oil: Stimulates scalp and improves blood circulation.
Rosemary & Fenugreek Oil: Promotes hair growth, repairs split ends and reduces hair breakage
Jojoba Oil: Manages frizz & flyaways
Jojoba Oil: Anti-ageing: reduces fine lines, wrinkles, and skin pigmentation
Description
Content goes here ..
Ingredients
Content goes here ..
Our Commitment
Our Commitment
What to expect
Content goes here ..
Description
Rosemary & Fenugreek Oil
- Chemical-Free & Safe – Suitable for all hair types
- Premium – Made with high-quality ingredients for hair growth and vitality
- Strengthens & Nourishes – Supports follicles, reduces hair fall, and promotes healthy growth
- Enhances Hair Health – Fortifies from root to tip for stronger, shinier hair
Jojoba Oil
- 100% Pure & Cold-Pressed – Rich in antioxidants and essential fatty acids
- Deep Hydration – Moisturizes skin, reducing fine lines, wrinkles, and pigmentation
- Versatile & Lightweight – Suitable for all skin and hair types
- Hair Nourishment – Promotes growth, repairs damage, and enhances shine
- Frizz Control – Tames flyaways for smoother, more manageable hair
- Ideal for All Uses – Perfect for both hair and skincare
Ingredients
Rosemary & Fenugreek Oil
Cold Pressed oils : Coconut Oil + Jojoba Oil + Almond Oil + Castor Oil + Sunflower seeds
Essential oils : Rosemary + Mint + Lavender + Fenugreek
Jojba Oil
100% Pure Jojba Oil
Our Commitment
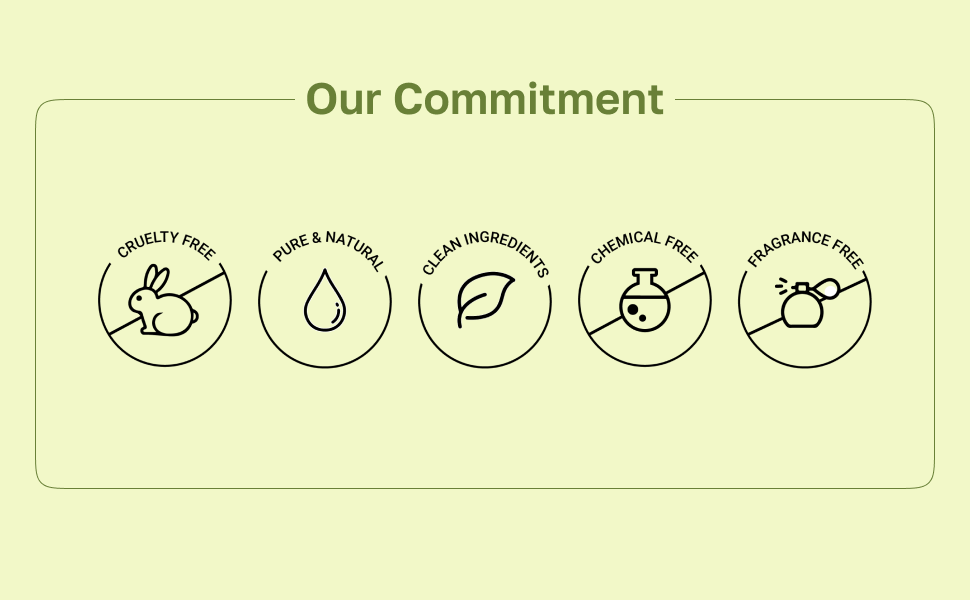
Why Choose Nurself?
1 . 100% pure & cold pressed
2 . Rich in Vitamins A, B, E, and Fatty Acids
3 . Deeply nourishes skin and hair
4 . No added preservatives 5 . Hexane Free
2 . Rich in Vitamins A, B, E, and Fatty Acids
3 . Deeply nourishes skin and hair
4 . No added preservatives 5 . Hexane Free
How To Use
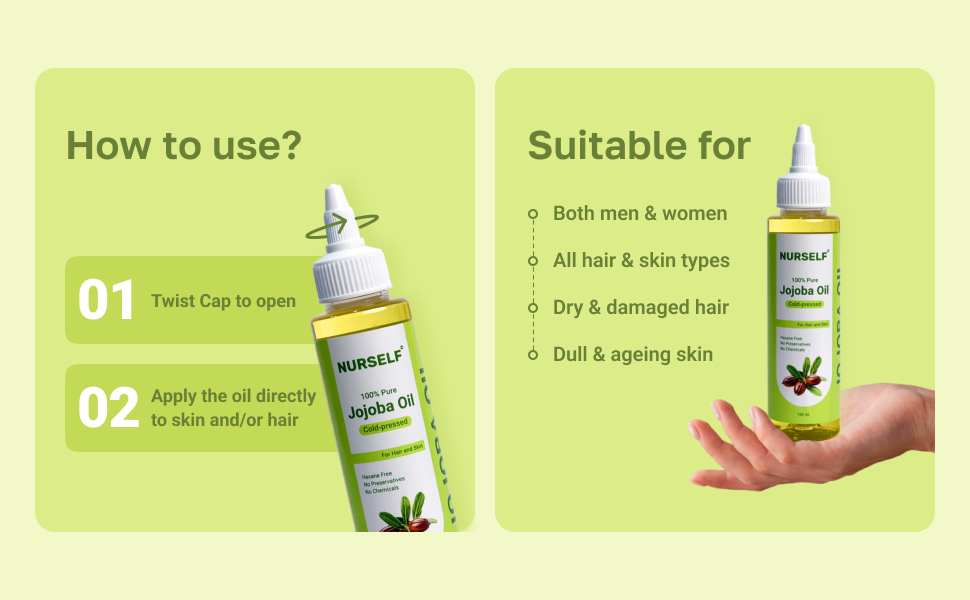
Reviews
There are no reviews yet